TL;DR; You can clone a Pokemon GO Plus device that you own. I have managed to get the certification algorithm. However, there is a per device blob used (specific to a Bluetooth Mac Address) for key generation. I have not figured out how you can generate your own blob and key. Using other’s people blob may be blacklisted in the future (or Niantic may ban your account).
Pokemon GO Plus, (which I will refer from now on as PGP) is a wearable Bluetooth Low Energy (BLE) device to be used with the Pokemon GO game for Android or iOS. There have been many attempts to clone this device, but only Datel seems to figure out the algorithm, while the other clones are cloning the exact hardware and firmware.
I will explain the complete certification algorithm that I obtain from reverse engineering a PGP clone, and then I will explain how I did the reverse engineering and how you can extract your own blob and key if you want to clone your own device. I am providing a reference implementation for ESP32 so you can test this yourself, the source code DOES NOT INCLUDE the BLOB and DEVICE KEY.
Before I begin, let me start with the current state of Pokemon GO Reverse and PGP reverse engineering.
A short history of past reverse engineering attempts
I am writing this to clear up some confusion that people have on the current state of Pokemon GO Game/App and PGP reverse engineering. The first few versions of Pokemon GO were not protected at all. In a short amount of time people were making bots and maps. Then things changed when Niantic implemented a complex hashing algorithm for the requests to their servers, but this too was quickly defeated with collaboration from many hackers.
Starting from version 0.37 on around second week of September 2016 (which is the first version that supports PGP), Niantic added a very complex obfuscation to the native code, and since then they have changed the hashing method several times, and lately also added encryption. Since the obfuscated version came out, only a few people worked on cracking the new algorithms. For a while, there was a group that runs a hashing-as-a-service with a paid subscription but since a few months ago they haven’t reopened their service. Either they have not figured out the latest protection or may be catching up with Niantic is getting boring or no that profitable.
On the PGP side, ever since this device was announced in 2016, many have tried to reimplement it in some form of hardware (for example this). Before the device was announced, the Pokemon GO app was still not obfuscated and the certification algorithm was not included yet. When the PGP device was finally released, the corresponding Pokemon GO app that supports it was already obfuscated.
On January 2017, a Reddit user BobThePigeon_ wrote a quite detailed article about reverse engineering PGP device. He figured out part of the certification process, but it turns out that it was not the complete process (this is the reconnection protocol). The certification is done at first connection which generates a key to be used at subsequent connections. His write-up only covers the reconnection part. Unfortunately, he didn’t continue this effort, and he never posted anything related to this. So currently (until now) there is no open source PGP device available since the device release data in 2016.
Even though no one has published the certification algorithm, Datel/Codejunkies has managed to reverse engineer this and released their clone: Gotcha and Gotcha Ranger. Just for your information, this company has been in this reverse engineering business for a few decades.
PGP BLE Peripheral
This background information is needed to understand the certification algorithm. PGP is a BLE peripheral that provides three services:
- Battery Level (a standard service)
- LED and button (a custom service)
- Certification (a custom service)
To be recognized by Pokemon Go app/game, it needs to announce its name as: “Pokemon GO Plus”, “Pokemon PBP”, or “EbisuEbisu test”.
Before the LED and Button can be used, we need to pass the certification process. There are three characteristics (“characteristics” is a BLE term) provided by the certification service:
- SFIDA_COMMANDS (for notifying the game to continue to the next step)
- CENTRAL_TO_SFIDA (for sending data to PGP)
- SFIDA_TO_CENTRAL (for reading data from PGP)
The flow of data is:
- The app can write anytime to CENTRAL_TO_SFIDA
- When PGP needs to send something, it sets the SFIDA_TO_CENTRAL characteristic value and notifies the app using SFIDA_COMMANDS notification
- The app can read anytime from SFIDA_TO_CENTRAL
I will not go into detail about the other two services:
- The battery service must exist because the app reads it
- Someone already reversed engineered the LED pattern, so I
wont go into detail about this
Certification Algorithm
Several people have tried reverse engineering based on the Bluetooth Low Energy (LE) traffic but were unable to get the detail of the certification algorithm. This is because the protocol uses AES encryption with a key that is not in the transferred data (so protocol analysis based on traffic alone is not possible).
First I will describe three special functions needed by the protocol. The first one is AES CTR. This is the same as normal AES CTR, except for the counter initialization and the increment function.
Please note that when exchanging nonce we exchange 16 bytes, only 13 bytes are used, and the other 3 bytes are overwritten. The nonce for AES CTR is prepared as pictured: first byte, and last two bytes are set to 0, and we copy the 13 bytes of the nonce (starting from offset 0) to offset 1 in the nonce.
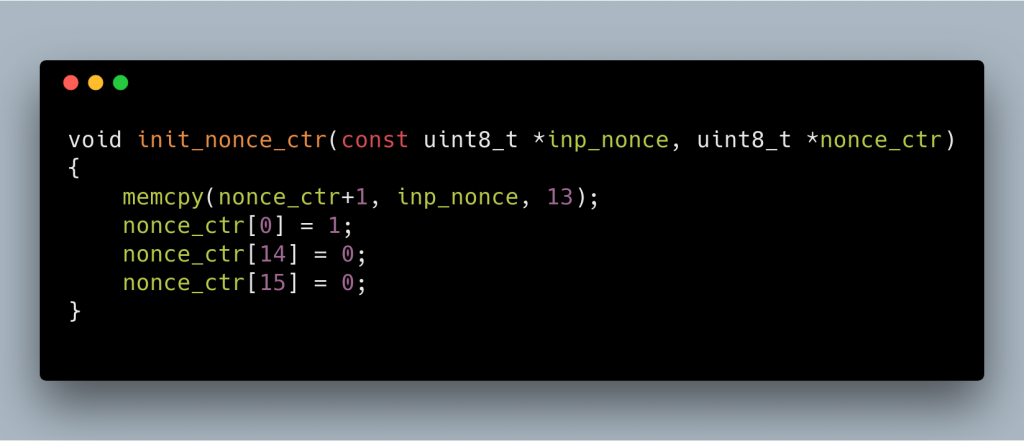
To increment the nonce, we increment the last byte (offset 15), and when it becomes 0, we increment the previous byte (offset 14)
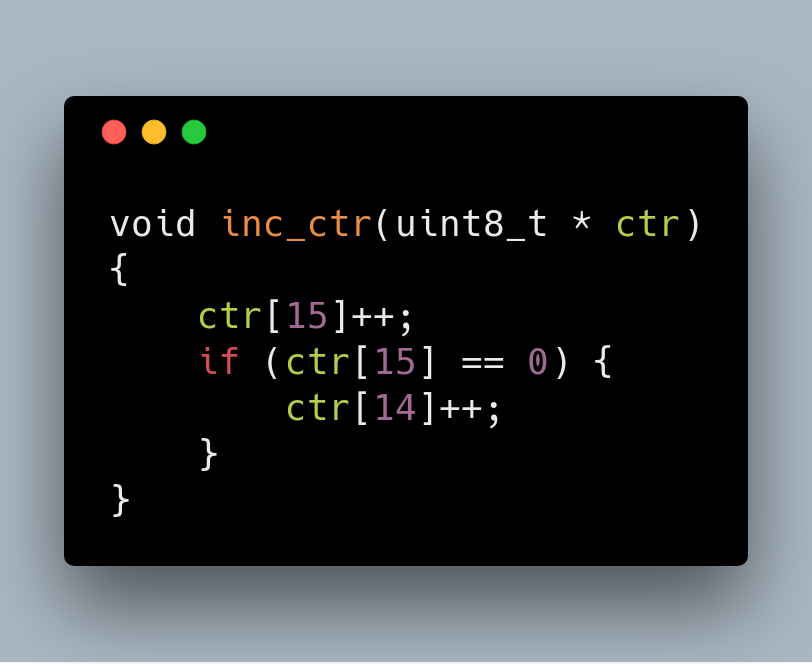
And this is how the AES-CTR is implemented
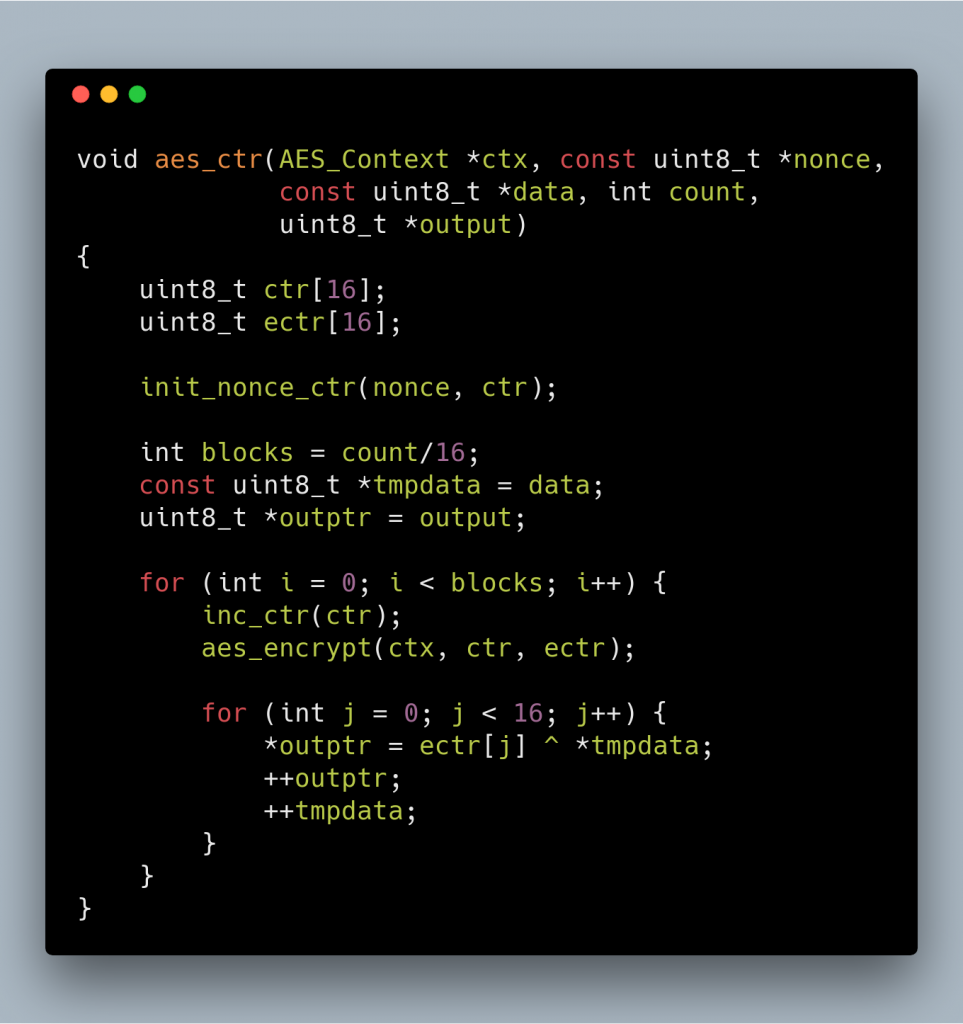
The second one I named it AES Hash, which uses AES to create a 128 bit hash from data. This one requires another nonce which is derived from nonce. This time we set the first byte to 57, and the last 2 bytes to the size of the data to hash.
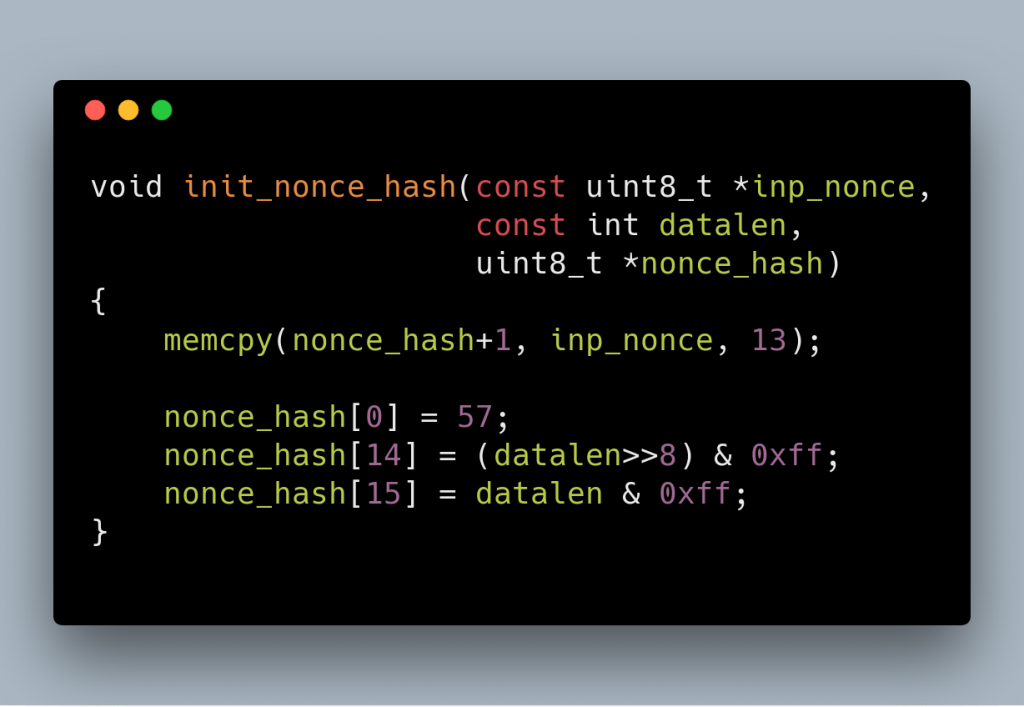
And here is the hash algorithm. This just encrypts the nonce, then xor it with each block of input data, then encrypt again.
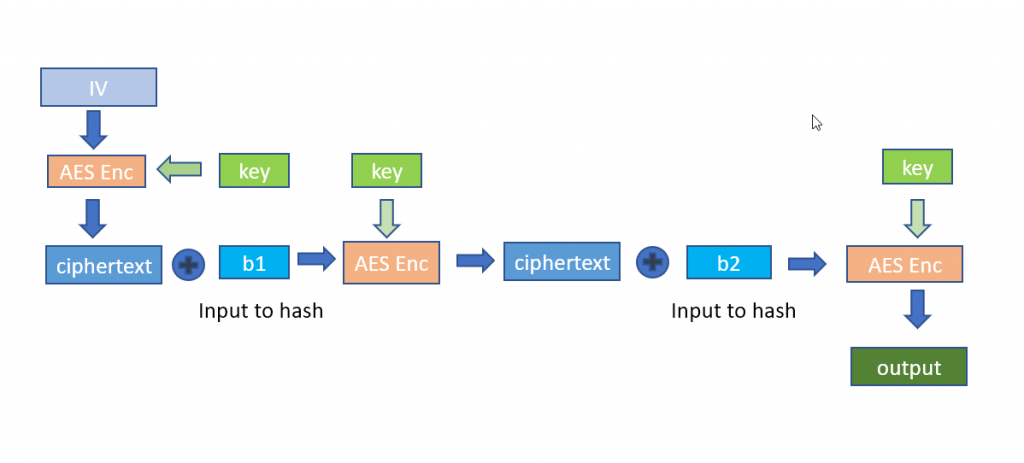
And the code is as follows
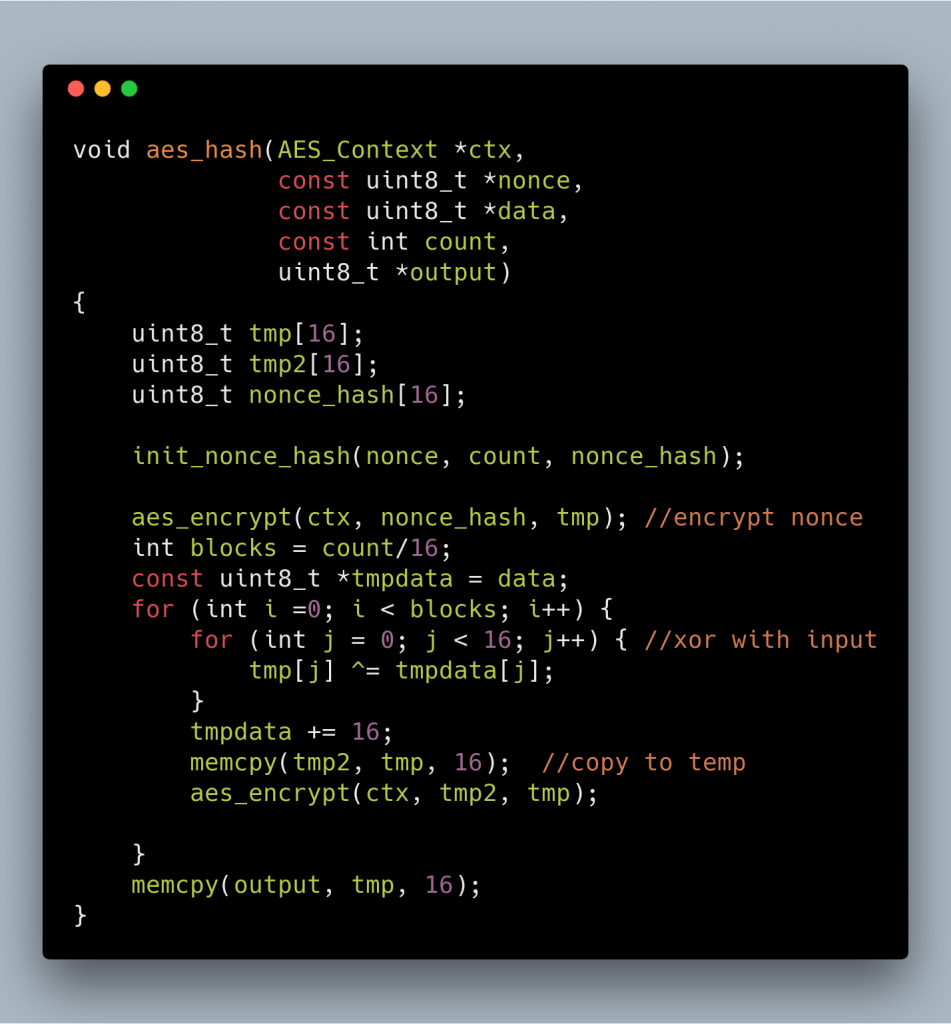
The third one is Encrypt Block which encrypts a nonce that is initialized as if it is going to be used in AES-CTR, then xors it with a data.
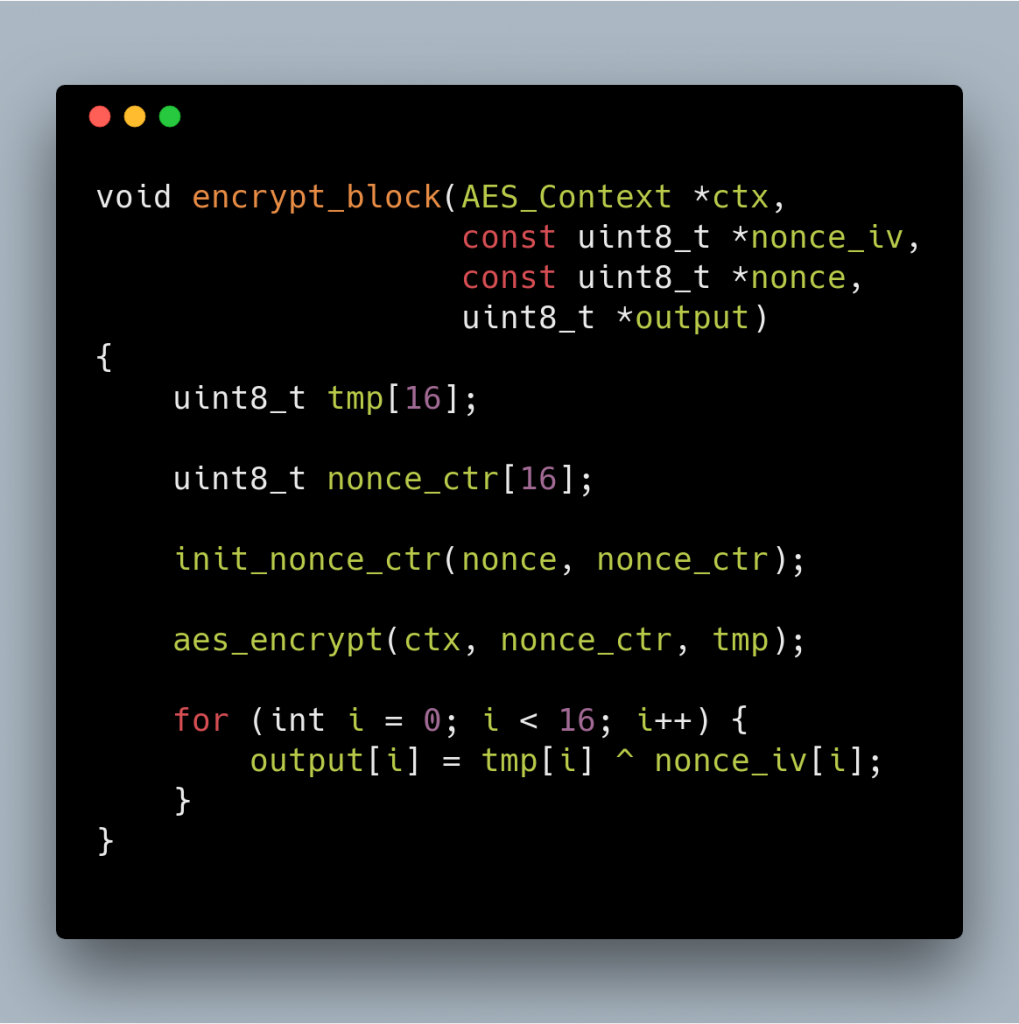
Now we can discuss the protocol.
PGP will generate a random 16 bytes challenge (A), a random 16 bytes session key (Sk), and a random 16 bytes nonce (N1). Encrypt A using Sk . This key, encrypted data, encrypted hash and nonce along with Bluetooth address (in reversed order) and some data obtained from the SPI flash (in my case it is always all 0) is then packed in the structure pictured below.
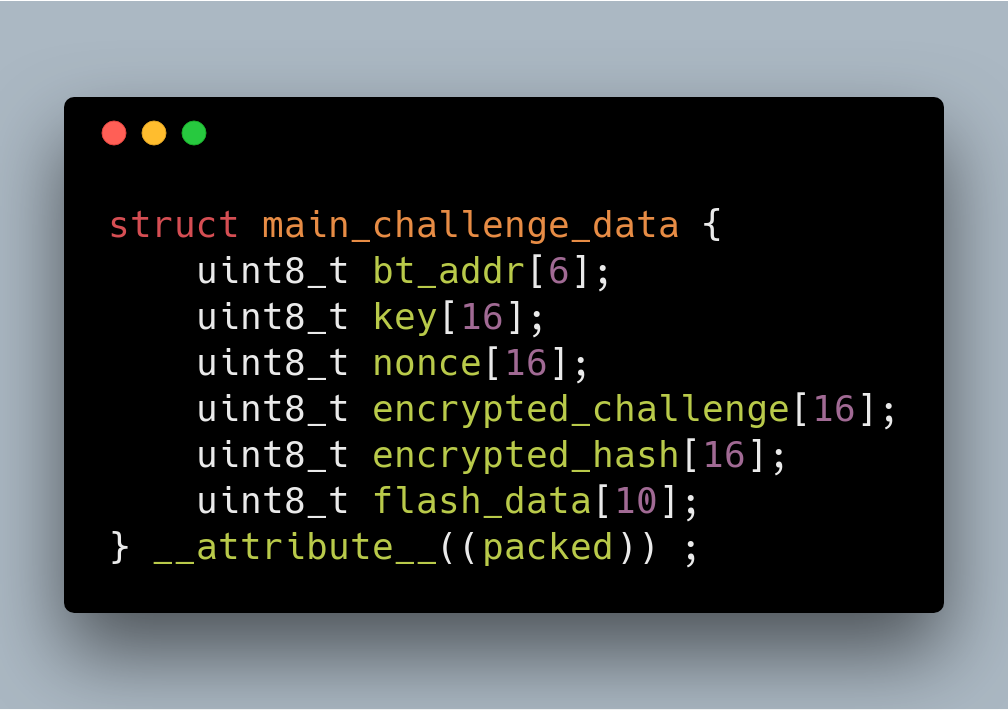
The content of encrypted_challenge is the output of aes_ctr. The content of encrypted_hash is result of aes_hash encrypted with encrypt_block. This explanation also applies to next parts wherever we have “encrypted_challenge” and “encrypted_hash”.
The 80 bytes is then encrypted using a “device key” which is dependent on the device. PGP prepares 378 bytes of data, consisting of:
- State (always 00 00 00 00)
- The encrypted main_challenge_data (80 bytes)
- A
nonce (this nonce can be different from the nonce inside main_challenge_data) - The encrypted hash
- Bluetooth Mac address (this is also on the encrypted main_challenge_data)
- 256 bytes of data blob from OTP (one-time programmable memory)
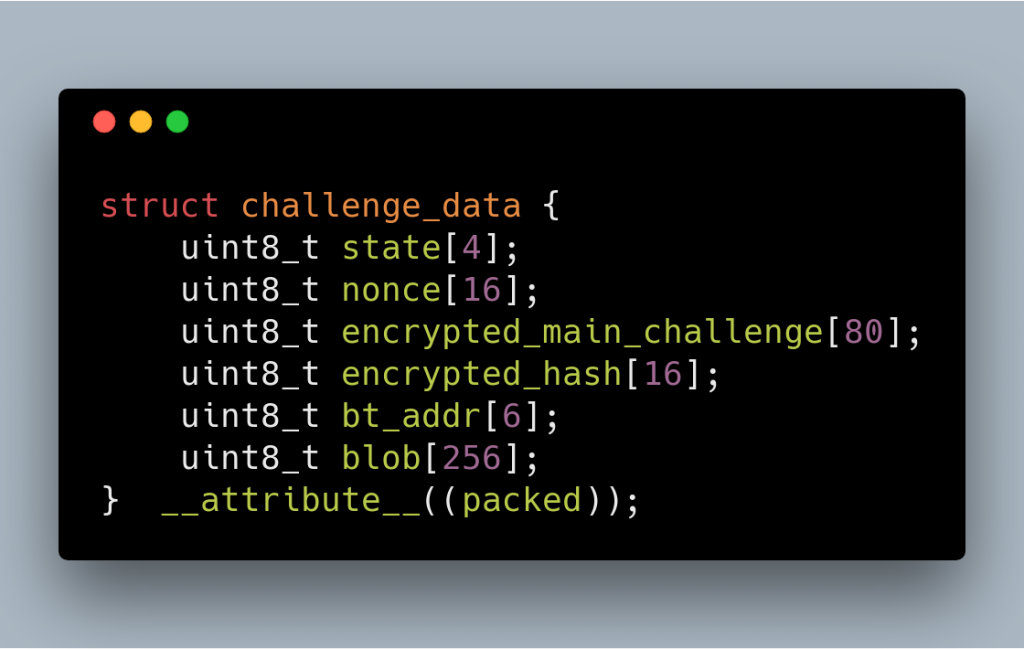
When Pokemon GO App connects to PGP, PGP will prepare the challenge data, then signals the
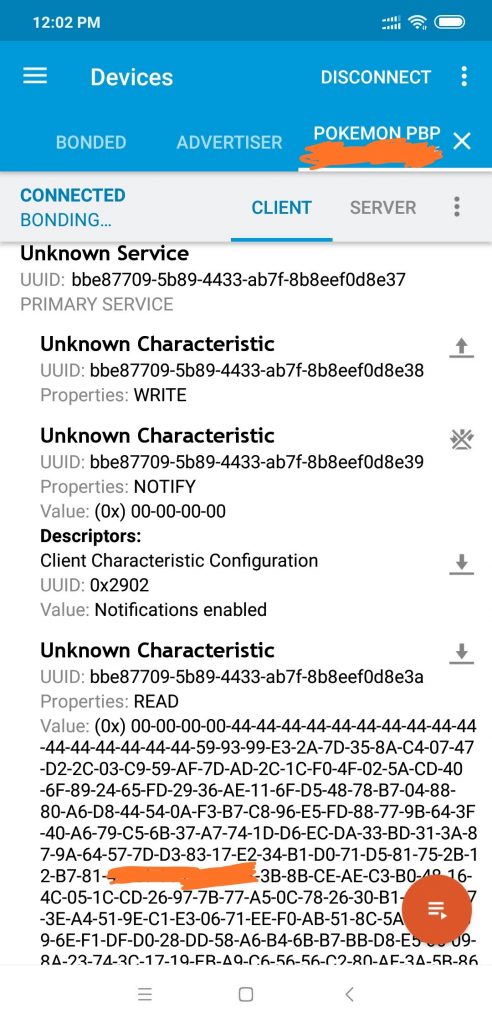
PGP will check that the 16 bytes (A) are indeed the same as the one that was sent. If not then it will terminate the connection. In general, in any step, if something is not right, the
For the next few steps, the challenge will have the following format (size of this is 52 bytes).
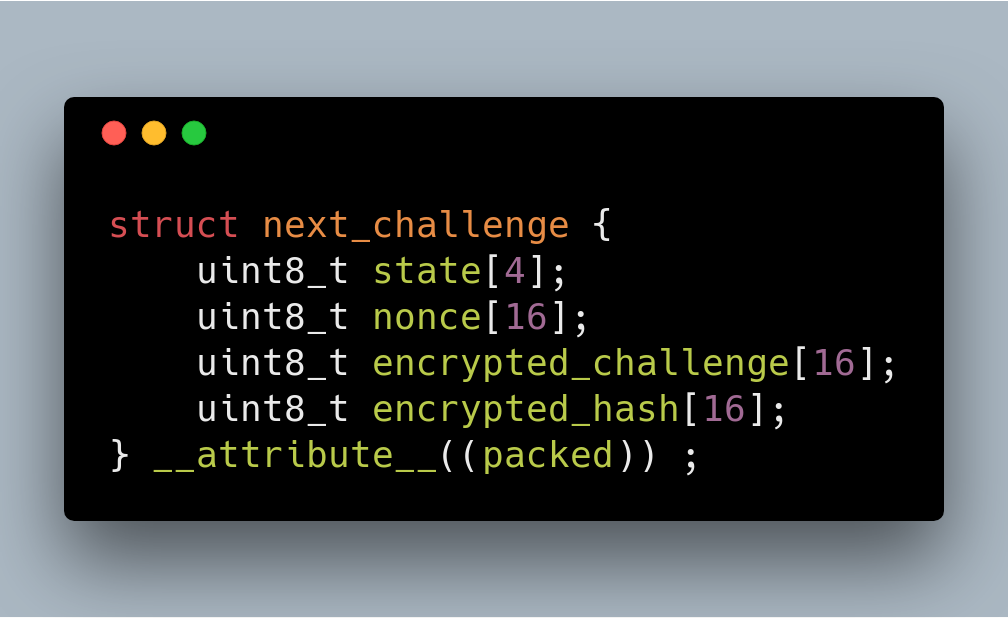
PGP will encrypt this static data: 0xaa followed by 15 bytes of NULs (0x00), using the session key (Sk), sets the state to 01 00 00 00 and notify the app to read the data.
The app will decrypt the data, and check if the decrypted data is 0xaa followed by 15 zeroes. If it is as expected, the app will generate random 16 bytes data, encrypts it and pack it in the same format. Note that in BLE world, app payload packet is limited to 20 bytes, so this will come in several packets.
PGP now needs to decrypt this data, and prepare a buffer prefixed by 02 00 00 00 and notify the app to read it. This proves to the app that the PGP device can decrypt the data from the
The app will send 52 bytes of data (again according to the next_challenge structure) then PGP will just notify with the value: 04 00 23 00,
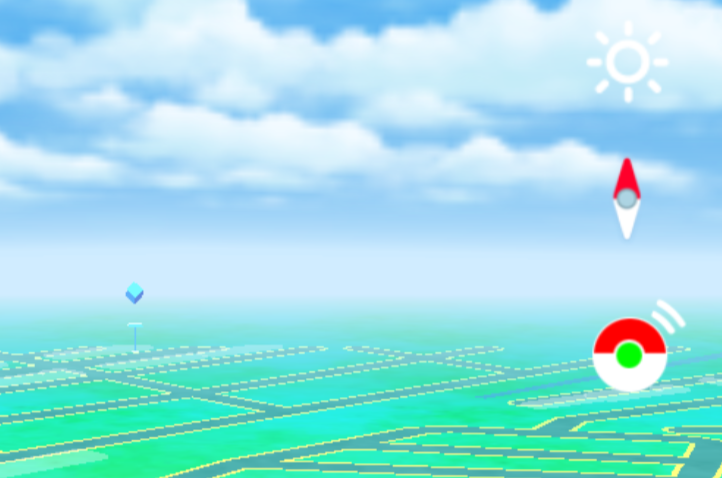
At this point, the green color should light up on the PGP icon.
We can tap on the green icon, and the app will disconnect from the PGP. We can tap it again to reconnect. At this point, we can just forget about everything and starts the protocol from the beginning again, or we can perform a reconnection protocol which is faster. This reconnection protocol is the one that is explained by BobThePigeon_.
For this reconnection, we will use the session_key (Sk) that we use in the previous exchange. PGP will generate two 16 bytes random value (let’s call them A and B), and expects the App to respond with:
AES_ENCRYPT(session_key, A) xor B
After PGP verifies that it is correct, the app will then send another two 16 bytes random (let’s call them C and D), and expects PGP to respond with:
AES_ENCRYPT(session_key, C) xor D
When the app verifies that everything is fine, it will send
03 00 00 00 01, and PGP will then acknowledges by notifying the value: 04 00 02 00.
LED, Vibration and Button
When we encounter a Pokemon or Pokestop, the app will send a pattern of lights to be played by PGP. The app will then read the button status to decide what to do with the information. So we can’t reprogram it to select a particular ball or to give berries.
Because other people have explained this better than me, I will not repeat it again. Here is a good explanation from a Reddit user on this thread.
GO Plus LED_VIBRATE_CTRL and BUTTON_NOTIF
Reimplementing the hardware
The next logical step after understanding the process is to reimplement this algorithm in a new hardware to test that it is indeed correct. I started with Android (turns out to be almost impossible), then Raspberry Pi Zero W (got stuck on some bluez stuff), and finally resorts to ESP32.
Android BLE peripheral emulation
At first, I thought that this is the best method: anyone that has a spare Android can test this. It turns out to be not easy: Android can act as a BLE peripheral but will randomize its MAC address on every announcement. This is done for privacy reason but I thought that it will make it impossible to implement PGP emulation because the PGP protocol uses Mac Address in the encryption process.
It turns out that on iOS, the app can’t get BLE address of the peripheral, so I (or someone) should try again reimplementing this in Android. For the Android game version: Niantic should be able to detect/block this easily.
Pi Zero W peripheral emulation
The next arsenal that I have is a Raspberry Pi Zero W. I have checked that it is possible to do peripheral emulation using
However, I was stuck with the bluez/dbus API. The documentation is quite sparse. So I gave up with Pi Zero W. I think it should be possible to do this in Pi Zero W. I don’t want to spend a lot of time debugging the bluez stack so I switched to something that is easier to debug.
ESP32
I chose this device because this device is very cheap (the cheapest is around 5 USD delivered), is easy to program, and I happen to have a few of them. I didn’t have any experience before in programming BLE for ESP32, but programming BLE in this platform is very straightforward. Please note that I just copied and modified the examples provided in the esp-
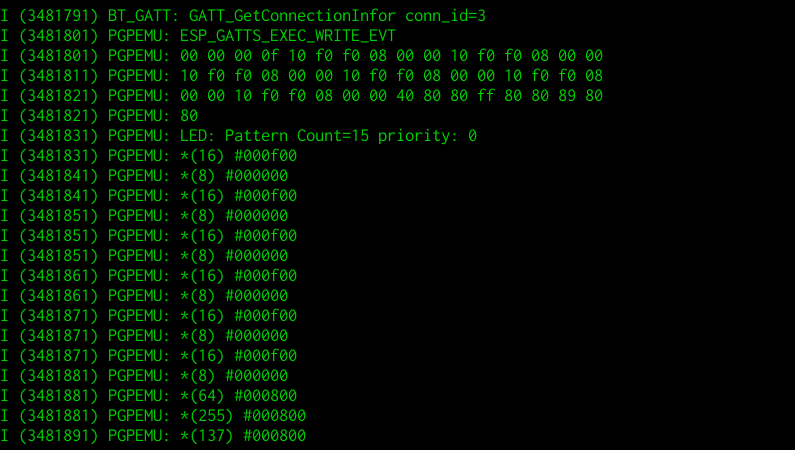
I also provided Makefile.test which can be used on the desktop to test the encryption algorihtms, just run make -f Makefile.test and run cert-test.
You can download the code from GitHub:
https://github.com/yohanes/pgpemu
This app doesn’t have a visual indicator, after flashing with “make flash”, run “make monitor” to see pairing progress. Press “q” to simulate button press and “w” to clear button press notification (although this doesn’t seem to be necessary).
I didn’t test the implementation for an extended amount of time. I only tested the following:
- It can be paired, disconnected, reconnected
- It can receive notifications when there are Pokemon around me
- It can send button press (using ‘q’ key in the serial monitor) to catch the Pokemon
You will need the following data from the device that you clone:
- Bluetooth MAC address (easily extracted using Bluetooth connection)
- Fixed data (easily extracted using Bluetooth connection)
- device key (currently requires soldering)
You can read the method to extract the device key on the next part.
Reversing PGP
This is the details of the reverse engineering part. This part is divided into two main parts: the hardware reversing and firmware reversing.
The hardware
I bought a Chinese clone of the PGP for about $20 including shipping (the original one would cost me $88 including shipping to Thailand)., and when I opened the PGP, it turns out the be an exact clone of the original. It uses the same DA14580 chip with the same PCB layout.
The first difficult part in reversing a hardware is to extract the firmware (since no one has shared this on the internet). To be precise: the difficult part is soldering the wires to the SPI flash chip. Information from BobThePigeon_ post helped a lot because I don’t need to figure out the pinout.
Fortunately, this one is a bit easier to solder due to the solder pads that exists in the cloned version.
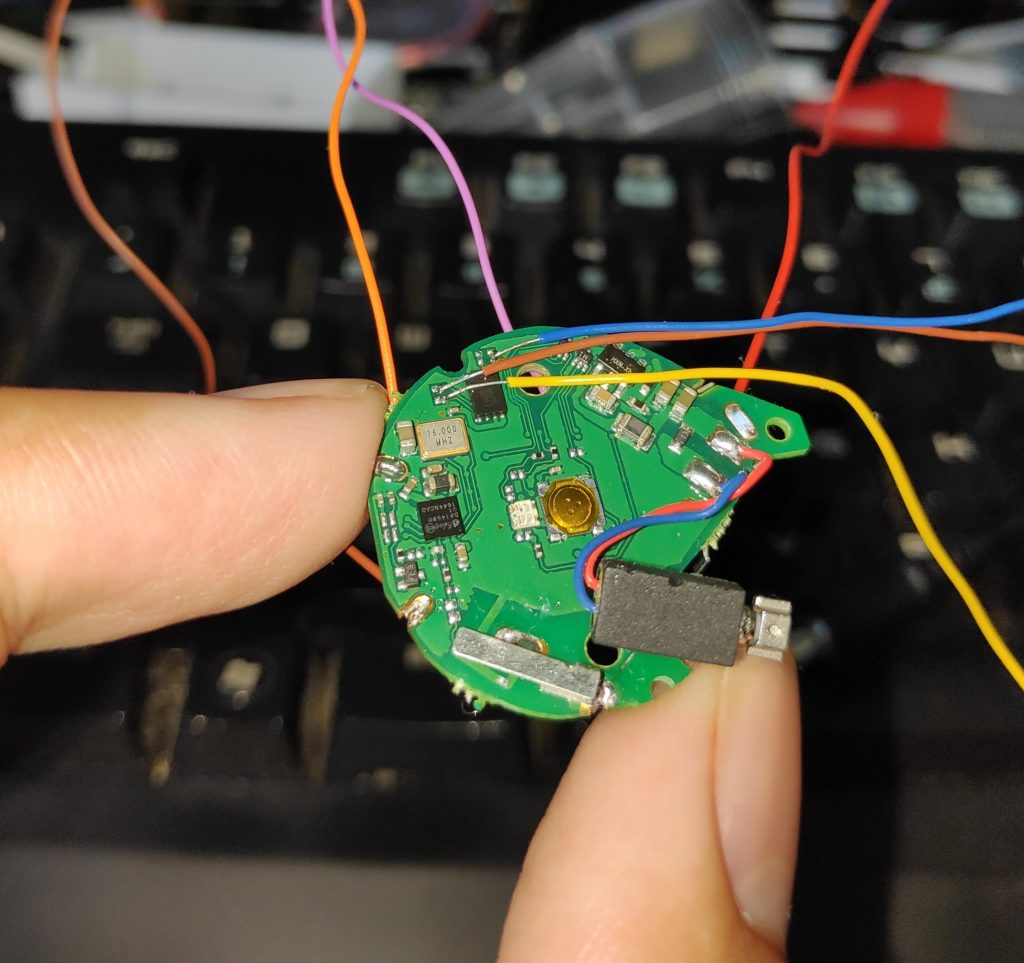
I used a $5 USB Soldering iron with a wrapping wire and it works quite OK. I was a bit amazed that the everything works on the first try.
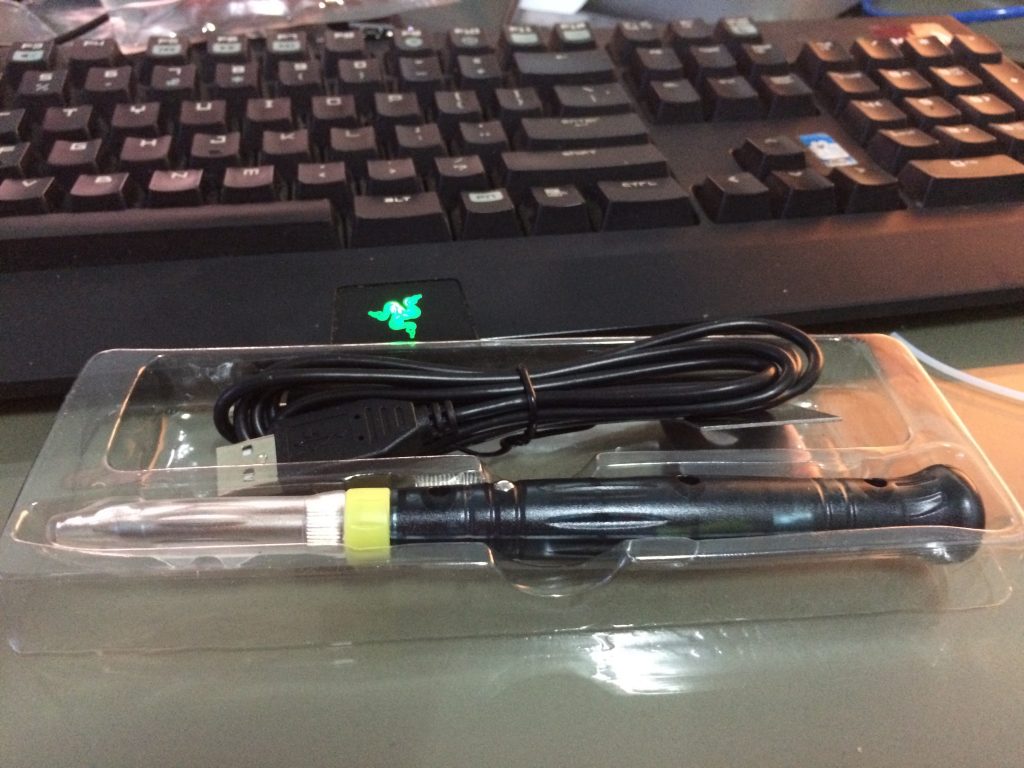
I held the board in place with K
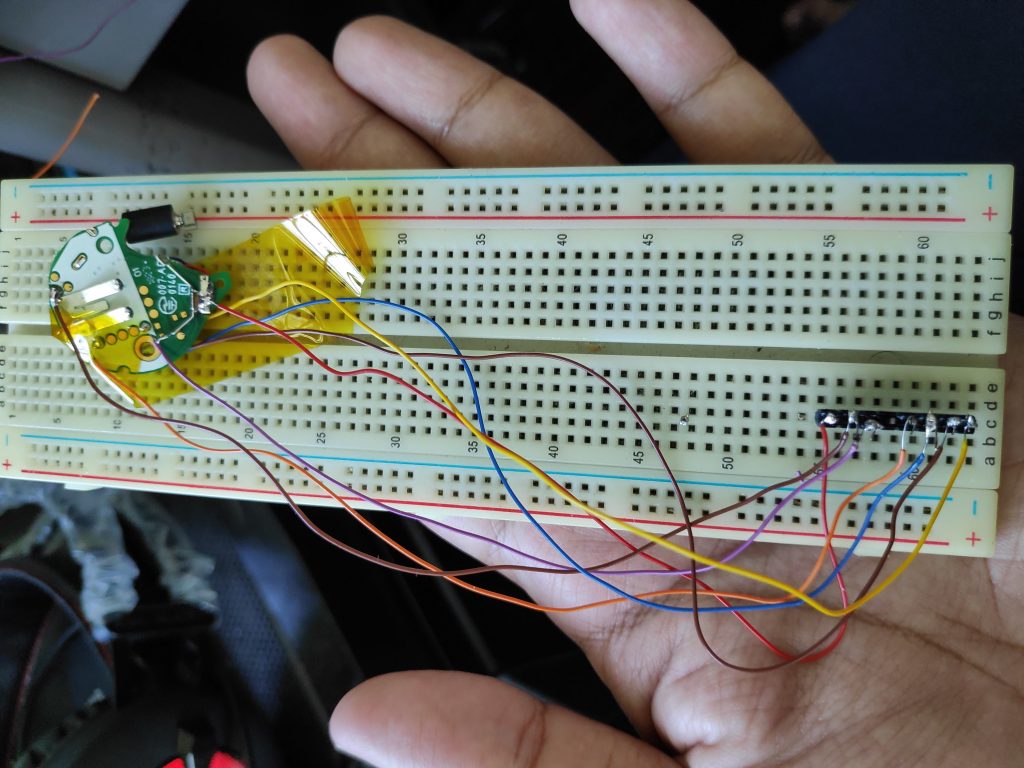
Since the hardware is the same, I tried following what BobThePigeon_ already did: holding the RST and read the flash. I use the
To detect if the SPI connection works:
flashrom -p linux_spi:dev=/dev/spidev0.0,spispeed=1000
To read the flash:
flashrom -p linux_spi:dev=/dev/spidev0.0,spispeed=1000 -r pogoplus-31-10-2018.bin
Later
After extracting it and comparing the description with his write-up, it turns out to be using the exact same firmware as described by BobThePigeon_. So all the AES keys that encrypt the firmware is also the same. It also means that when there is a new update for PGP, this device should also be updateable.
For the hardware debugging, I did not use JTAG to debug the firmware. I did not perform a dynamic analysis using a debugger. So basically I only used a few cables to read and write the SPI flash.
Deeper into the firmware code
BobThePideon_ wrote that “All this information is in Dialog Semiconductor’s DA14580 SDK, however, you have to jump through some hoops to get the SDK.“. Well, it turns out getting the SDK is quite an easy process, and having the SDK helps a lot in understanding the firmware. Even though I don’t have a DA14580 devkit board, I can try to compile and see what the resulting code will look like.
The main firmware is 31984 bytes long and since this is Cortex-M0, it uses Thumb instruction set. This firmware is loaded at starting memory location: 0x20000000. It is not easy to understand the code just by looking the code in a disassembler, so my first approach is to try to see what a real firmware would look like if it has a full debugging information.
After installing the DA1458x_SDK and Keil uVision5 we can compile the examples (for instance ble_app_all_in_one). My first thought was to generate assembly code from C file (like the -S option in gcc), but this is not allowed in the free version of Keil uVision. But we have the next best thing: an ELF with debugging symbol. On the output folder, I saw an AXF file, which is actually an ELF file with debug information. This helps a lot in understanding a firmware designed for DA14580.
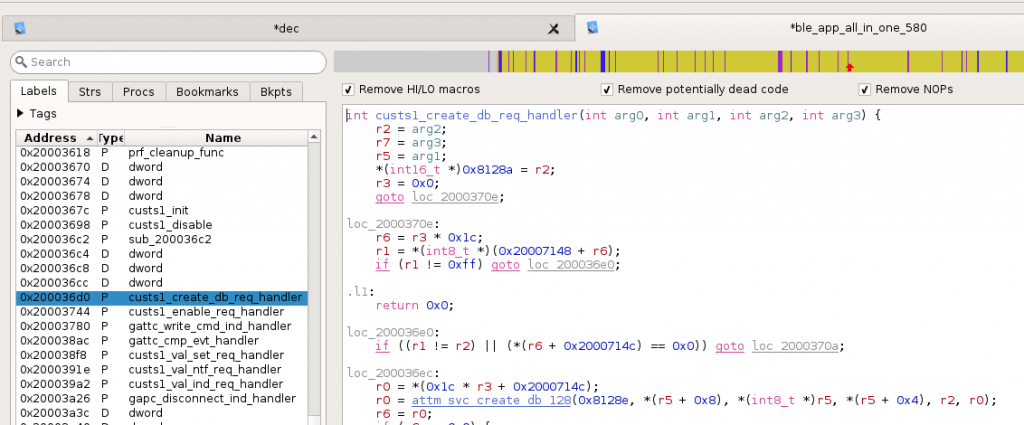
Now we can see clearly how the code calls ROM functions which is located from 0x20000-0x35000, and I can understand the mapping in rom_symdef.txt file:
sdk/common_project_files/misc/rom_symdef.txt
For example, in the PGP firmware, the function at the address 20006e24 just calls 0x33b21 which according to the rom_symdef.txt is __aeabi_memcpy. Renaming these procedure is like finding the edges of a puzzle.
I spent quite a lot of time looking at SDK to understand more about its structure, convention, and constants that might help.
Inside platform\core_modules\rwip\api\rwip_config.h we can see the standard TASK number (for example for battery service, firmware update service, etc). This task numbers also helps identify different subroutines that call ke_msg_alloc,
Some interesting subroutine/function locations:
- At
address 0x20005758 is the function that copies data from OTP (blob and device key) - At address 0x200065DC is the main AES encryption (also the read AES implementation in sdk/platform/core_modules/crypto/), by finding cross-reference to this, we can get the subroutine that does AES-CTR, AES-HASH, etc
- At address 0x2000644E is the handler that will handle different states of certification
The rest is just patience to trace every input and output of the subroutine to see how each value is generated. After reading things very carefully for a couple of days, I figured out all of the algorithms. It took me another few days to track the key being used, it turns out that the device key is not stored in the SPI flash but in the OTP (one time programmable) area (starting from 0x47000). The blob is broadcasted on the challenge, but the key is not.
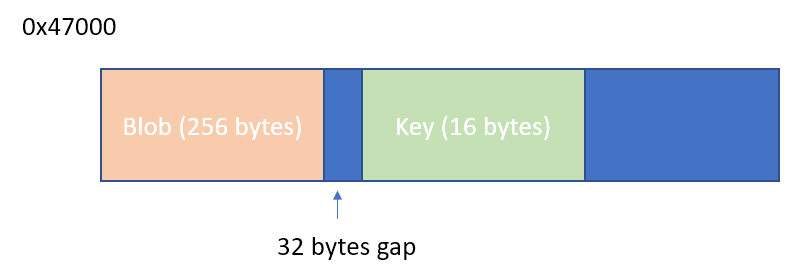
The secret recipe (or how to extract your key)
So the code uses some data from the OTP area of the chip for the device encryption key. How can we read this? there are a lot of ways to do it:
- Write a new code to read the OTP area
- Use a debugger
- Patch the existing code
Writing a new code or using JTAG debugger will require me to solder more wires and to get the debugging tools to work. I am trying not to disturb the current soldering that I already made, and I don’t want to setup environment o write a new code, flash it and read the result.
I found a simple way to extract the key by patching a single byte on the firmware. I just shifted the constant that was used to send the blob. Instead of sending only the blob area, it will send also the embedded key that is located 32 bytes after the blob.
Compared to JTAG method, this patch is easier to reproduce by anyone that has a soldering iron and Raspberry Pi or Arduino.
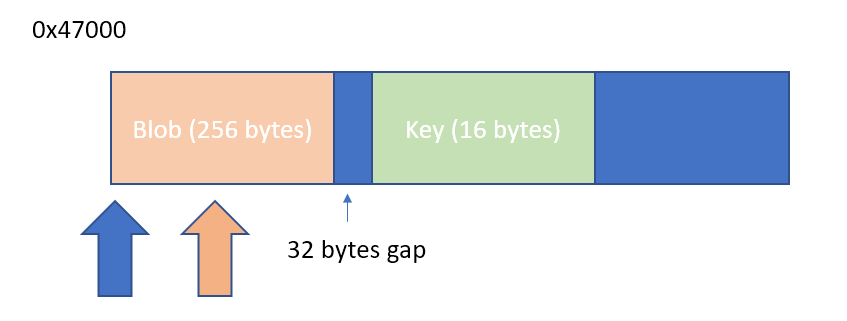
This is just a one-byte patch, by changing the value at 0x6425 (file offset in the decrypted main firmware) from 0x4c to 0x7c, we can extract the device key.
I provided a script to decrypt firmware from SPI flash image, and also another script re-encrypt the decrypted firmware to make a new flashable image. So the steps to get the keys are:
- Get the SPI flash content
- Extract/decrypt the main firmware
- Patch the main firmware (remember to make a backup of the original)
- Repack/re-encrypt the patched firtmware
- Flash the firmware
- Start the PGP
- Read the challenge data using any software, the last 16 bytes is the device key
- Flash the original firmware
Is there another way to extract the device key? It may be possible to extract it from the memory of the Pokemon Go app while it is running. But even if it is possible now, Niantic may change it so that extraction will not be possible in the future (e.g: by clearing keys after use or even do the decryption on the Niantic server).
Reversing the Pokemon GO game?
I tried reverse engineering the Pokemon GO game, but it is heavily obfuscated. I spent a few hours a day for several days but didn’t get very far. I can explain some of the things that I observed, but I won’t go into much detail since Niantic will probably change them anyway.
Before going deep into the code, I checked on the version history of both the APK and IPA files, hoping that may be in the past they have included the certification process in an unobfuscated form (or less obfuscated form compared to what we have now).
All versions before the release of PGP hardware contains incomplete PGP certification code which is still not obfuscated. Unfortunately, both the iOS and Android version contain obfuscation at the time of the PGP release. The old files are also not easier to read compared to the newer ones.
iOS version of the game
The iOS version consists of a single huge binary named pokemongo. This is a mix of Objective-C, Unity and native C++ code. The binary uses ARM64 code. Due to the size, navigating and extracting something useful from this monolithic binary is quite hard.
There is a group that actively maintains a patched version of Pokemon GO called PokeGO++ (they even have a subscription for this service). They patched the security checks that exist in the binary and added a new library with method swizzling to add new features to the game (such as Teleport, IV Checker, etc). If anyone is interested in reversing the Pokemon GO game, then this would be a good starting point.
I did not investigate the iOS version further, apart from the big binary size, the other reason is that I only have an iPhone 5S which is already too slow to run the Pokemon GO game.
Android version of the game
In the Android version, Niantic employs SafetyNet so that changing the APK will stop it from working. It is also very sensitive to any leftover trace of rooting tools, and the existence of some files will make it refuse to connect.
The Android version consists of Java/Smali Code, Unity code, and native library (accessed through JNI). Using existing tools we can decompile the Java part, but nothing interesting is there. We can also look at names of the Unity classes using existing tools, but the implementation is in native code (not in .NET IL). The native library uses THUMB instruction set instead of ARM/ARM64 (even on 64 bit Android).
Almost every subroutine in the native code is split into tens to hundreds of blocks. My guess is they are using a custom obfuscating compiler, probably a fork of llvm-obfuscator. One subroutine is split into multiple blocks using MOV Rx, PC. This can be fixed using some pattern matching, but after you merged the routines, it turns out that it is still split into multiple small subroutines located far away. This small subroutines only does one thing, for example a+7 or a + b.
Strings are encrypted (obviously), but the decryption is not done in a single place. It is done when needed, and it also uses a different encryption method in each subroutine. So string extraction is not easy.
Although I didn’t implement it, in my opinion, it is possible to
The native code is accessed using JNI, but it only exports several symbols named java_XXX, for the rest, it uses “registerNativeMethods“. Of course, the address and the name of the methods are obfuscated.
The process for PGP is separate from the game process. The game communicates using SSL. Bypassing the SSL is not too hard, but apart from the initial handshake, subsequent packets are encrypted with custom encryption (they use another layer of encryption on top of SSL).
For the main game process, we can intercept the Unity code to see the request/response in Protobuf form, but unfortunately, this is not possible in the PGP process which is completely native code (it doesn’t load the unity library at all).
During the pairing process, the Pokemon Go game must be connected to the internet. This seems to indicate that whatever process required to generate the key was done on the server (Niantic) side.
Reversing other devices?
Currently I do not own any other Pokemon GO related devices such as Poke Ball Plus, Nintendo Switch or other implementation of PGP (such as Gotcha, Gotcha Ranger, and Pocket Egg) so I leave it to others to do it, or I might do it when I have the device(s).
One interesting thing is that we can rename the device to Pokemon PBP and it will be recognized and paired as Poke Ball plus.
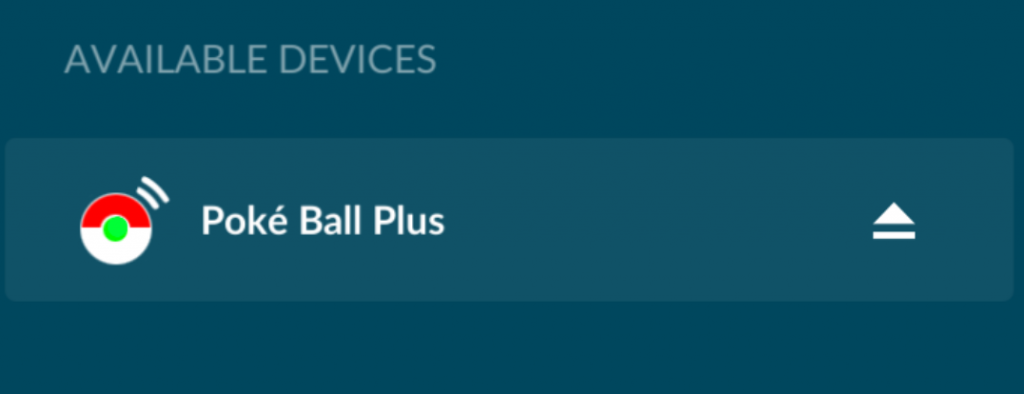
How can datel/codejunkies and the Chinese do it?
The short answer is I don’t know. I don’t know how they can generate the combination of a new blob, mac address, and device encryption key.
Changing a byte in the blob (with same Mac) causes the challenge to be rejected. Changing a byte in the mac address also causes the challenge to be rejected. I only have one device to test and although I have many guesses, I am not sure which one is the answer.
Some of my speculations are:
- There are only a few combinations of Mac/Blob/Keys in the cloned devices being sold (as noted by many people, it seems that most/all Gotchas have the same MAC address), or
- There is a simple formula relating these three, and it doesn’t use any secret key, or
- There is an implementation of this algorithm in one of the old version of the game, or
- The algorithm was leaked from the PGP factory
Or the explanation could be very different from the one listed above. I am also considering to release my Blob and Key, but I am afraid of these:
- Niantic might block this Mac Address from connecting (a bit unlikely, since they seem to allow Gotcha devices with same Mac address, but of course they can always change their mind)
- If this mac address is used by many people, everyone will be blocked
- I might get sued for publishing the secret key
But for now, I decided to play safe.
If someone wants to sacrifice their PGP and spread the blob/key combination, I suggest to use an original PGP, so that the Mac address is unique. And if that gets banned, you won’t upset a lot of people buying cloned devices.
Please also note Niantic’s stance on this.
What can you do now with this information?
There are several legal things that you can do with the information presented:
- You can clone your own device, and make it better (e.g: in a better form factor, with a better display, battery, etc). Cloning your own device for your own use should be undetectable by Niantic.
- You can modify the firmware of your PGP (e.g: auto catch or auto spin only)
- You can write an app that can communicate with your PGP
Future work
I only played Pokemon GO casually with my family, I am still at level 33 after two years playing this game on and off. Reversing this Pokemon GO Plus is only for fun and to satisfy my curiosity. But I am not that curious to spend a lot of money to acquire other kinds of Pokemon Go related hardware (Gotcha, original Pokemon Go Plus, Poke Ball Plus, etc).
If you want to help me buy other Pokemon GO related hardware or just tip me for this article, you can send it via:
- Paypal
- Bitcoin (19mkof1of9yC5TNWbPw5gjGrcL2NHiHim9)
- Ethereum or other tokens (0x618b59AF01DC11b7fBb00f700E9b78A5cc2e234e)